Looking to enhance the user experience on your website? Thumbnail hover effects are a great means of doing so.
A hover effect is an animation, image, or other effect that appears when you hover over an element on a web page. They can be used to convey more information, or encourage an action or encourage engagement with that element. Because of this, they enhance the interactiveness of a site for a more dynamic, engaging experience.
This article will guide you through the key methods and tools to use to effectively implement hover effects on your website. Once you’ve finished reading this tutorial, you will be able to use hover effects to improve the quality of their website, increase engagement, and improve conversion rates.
Exploring Different Types of Image Hover Effects
There are various image hover effects that you can use to enhance your web design, from simple hover effects like color transformation to more advanced animations for serving different users’ needs. Hover effects can include:
- Highlight/brighten image: this can bring an element, such as a background image, more into focus.
- Gray to color: if your images are left in grayscale, hovering can then colorize the image (or vice versa).
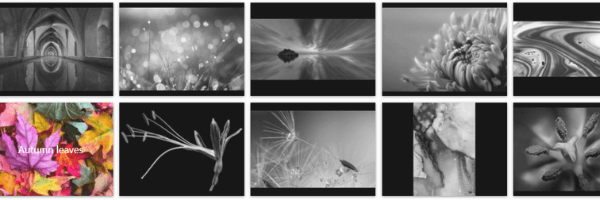
- Fading in/unblurring an image: you can blur or sharpen your image.
- Enlarge image shrink image
- Square to circle/circle to square: change the shape of an image on hover.
- 3D pop-out shadowing: With this effect, an image pops out while a shadow appears behind it.
- Add border to image
- Hover Animation: Your effects can be animated – such as a caption sliding with a change of color gradient.
Certain hover effects, such as highlighting, are intended for ease of use, by making it clear where on a page a user is. Other effects, such as zoom or pop-up are best for displaying and emphasizing certain information. In this way, hover effects can assist in the effective presentation of key information.
Other effects are mainly visual, such as adding color to a gallery of gray images. While this doesn’t provide additional information to the user, it does make for a more interactive and engaging user experience.
Here’s an example of how hover effects can be used to convey information and add visual appeal.
Choosing your effects strategically is crucial. These effects should not be added without intention lest your site lose clarity, or become overwhelmed with visual effects. Tools which have a variety of high-quality effects to choose from are thus especially helpful.
As Tam Vincent from FooPlugins notes: “Hover effects can change how users interact with a website. When used well, they can make things easier to understand or more fun – for instance, showing a button is clickable when you hover over it. But too many hover effects or using them for no reason can confuse people. It’s important to use them carefully, so they help users without making things messy or hard to use.”
Implementing Hover Effects More Broadly with Simple CSS Techniques
CSS (or Cascading Style Sheets) is the language used to style or design web pages. Essentially it is used to control how HTML elements are formatted and presented on your website. For example, CSS can be used to control color, font size, header size, or padding. More importantly for this article, CSS can also be used to create hover effects.
These effects can be implemented on a website by adding CSS within the HTML file or as an external stylesheet file. In WordPress, this can be achieved by adding the CSS to the WordPress customizer, the theme editor, the child theme, or by using a specific CSS plugin. And to make these changes, you would use the CSS selector :hover and then add your changes after that. (For a more detailed guide on how to implement CSS image hover effects, you can take a look at some of the trusted online repositories such as Codepen’s demo CSS hover effects.
Some of the more common CSS properties used in hover effects include:
- Color: You can change the color of an element, such as the font or a button, on hover.
- Opacity: This will make an image more or less opaque – if used on an image with captions, this effect can help the image stand out.
- Scale: With scaling, an element on the page can increase (or decrease) in size when hovered over. This gives the impression of zooming in.
- Transition: Here, the style or property of the element changes smoothly on hover. With a transition effect, a button may get darker, change color, and/or change font color, drawing the user’s attention.
There are some potential pitfalls when adding CSS to your WordPress website. There could be conflicts with the CSS used by your theme or other plugins or ,if your custom styles are loaded before the theme styles, they might get overridden. To solve this, you can ensure that your custom styles are loaded after the theme styles by using the wp_enqueue_style function. And check for JavaScript errors in the browser console and resolve them by modifying your scripts.
Another big concern is that hover effects designed for desktop may not translate well to mobile or other touch-based devices. You can accommodate for this by applying different styles for various screen sizes.
Regardless, this can be time-consuming and frustrating, especially if you don’t have a lot of experience with using HTML or CSS. Alternatively, it may be easier to use a plugin to support this process and ensure a professional finish (without the hassle of doing it all yourself).
FooGallery: A Simple Way to Add Hover Effects to Image Galleries
This is where FooGallery comes in. This plugin helps you create organized and professional image galleries, and includes specific functionality helping to create and manage sophisticated hover effects for image galleries. These effects are specific to image galleries, helping you ensure your images catch your visitors’ attention.
With FooGallery, you no longer need to worry about the burden and technical knowledge required to add hover effects manually. It is a user-friendly plugin and does not require technical expertise.
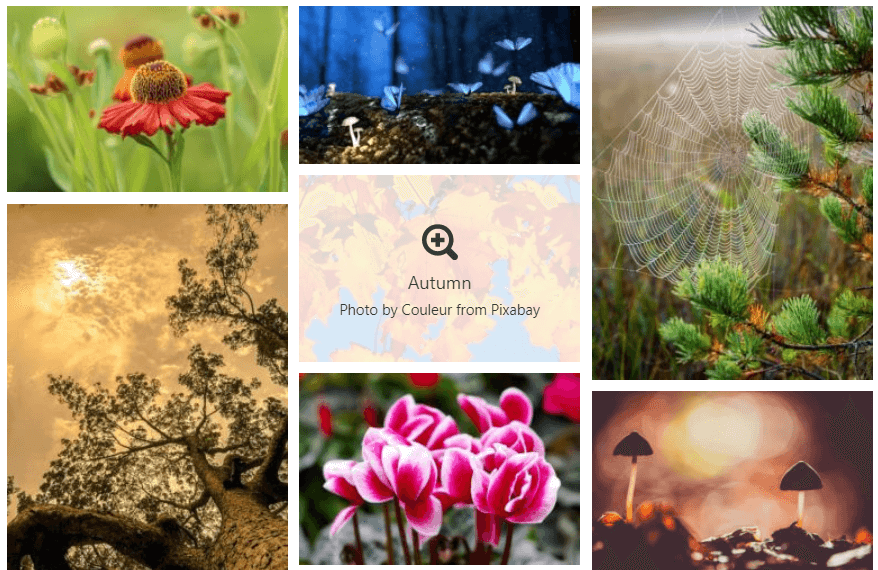
It’s also important to note that the more you add to your website, the more difficult it is to run. Adding hover effects, if not done properly, can actually slow down your website, which will negatively impact not only user experience, but even the SEO ranking of your site. FooGallery, however, is a lightweight plugin, which means that it will create minimal bloat and avoid any negative impact on your site.
There are various types of hover effects achievable with FooGallery, including icon pop-ups, zooming, box-shadows, fading, and overlays. Each of these hover effects can heighten the user experience on the website in its unique way, while also providing visual cues and information for the user. Such as a ‘plus’ icon to encourage visitors to click on an image, or having captions appear on hover to explain or describe an image.
With the FooGallery PRO Plans there are also hover effect presets available for ease of use. This gives you a further 11 aesthetic hover effects, all designed to highlight your image galleries. For example, Sadie which allows you to unfold a caption from an image title on hovering, while Selena scales the image and reveals the description.
Sadie…
Selena…
FooGallery also supports customization, giving users the freedom to tweak the hover effects to suit their specific design preferences and website needs. This might include a light or dark overlay, or a scaled, zoomed or colorized effect.
FooGallery’s functionality extends beyond hover effects to helping you create and organize gallery image and video layouts. As a first step, it is important to ensure that your images are of a high quality, something FooGallery supports through features such as retina ready images or improving thumbnail quality. The plugin supports you through the full process of adding images to your site, and then enhancing them with effects. It also integrates with other plugins such as FooBox, a lightbox plugin, to further enhance your site.
With the different plans available to you, FooGallery can be scaled to larger and smaller sites for the specific needs of your business.
The Best WordPress Gallery Plugin
FooGallery is an easy-to-use WordPress gallery plugin, with stunning gallery layouts and a focus on speed and SEO.
A Brief Guide on Using FooGallery to Set Image Gallery Hover Effects
FooGallery is easy to use and does not require coding skills, but still allows customization for advanced effects. So let’s take a look at how to use FooGallery to set hover effects.
- The first thing you will want to do is install FooGallery from the plugins page.
- Once activated, click on Add New under the FooGallery menu in your WordPress dashboard. In the new gallery, you can Add Media and select the gallery layout, or template, you want to use. Quick tip – if you want to see how your gallery appears as you make changes to the settings, toggle over to the Gallery Preview tab in the Gallery Items panel.
- You can now apply hover effects or hover presets in the gallery settings.
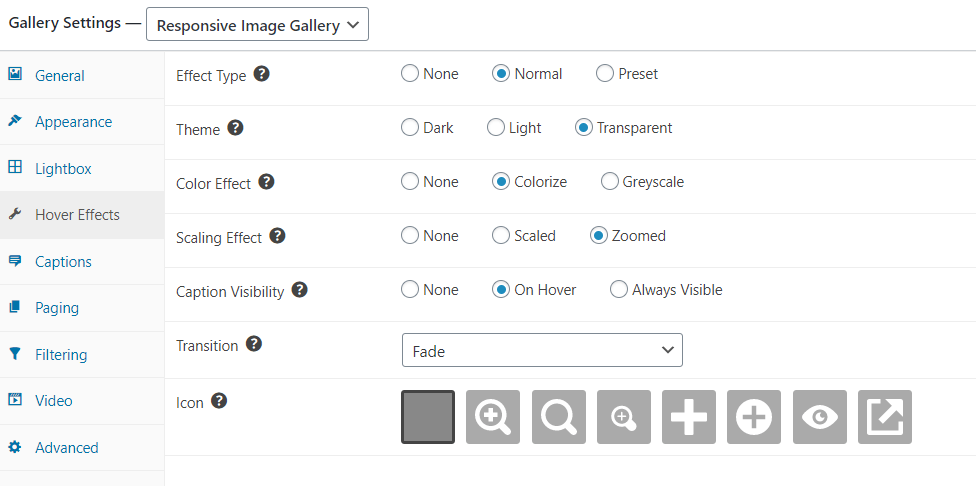
For more detailed information, take a look at the complete step-by-step guide to adding hover effects using FooGallery. But here’s a quick look at some of the various hover effects available with FooGallery PRO.
- Choose between a normal effect type or none.
- Choose between a dark, light, or transparent theme.
- Add a color effect to your hover. Here you can opt to have your thumbnails in Greyscale and colorize on hover, or vice versa.
- Choose a scaling effect. Make thumbnails scale slightly on hover, which increases the size of the thumbnail. Or Zoom on hover, which increases the image size within the thumbnail.
- Choose whether you want captions to remain visible on hover.
- Select a transition effect when you show the caption on hover. These include Fade in, Slide Left or Right, Push, to name a few.
- Choose if you want to set an icon to show on hover, and choose from several icons.
- Alternatively, with FooGallery PRO, you can opt for one of the hover presets, giving you a more advanced, stylized effect, minus the hassle of formatting the hover effects yourself.
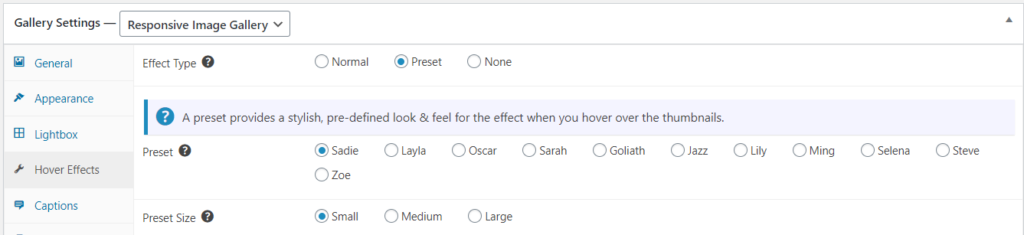
As you can see, FooGallery will streamline and facilitate the process of adding hover effects to your site, which can be difficult and lengthy. It will ensure an aesthetic, reliable, and professional finish to your site’s effects.
Best Practices for Implementing Hover Effects
Using hover effects is an effective way to draw attention, highlight elements on the page and provide additional information for your users. However, you should be aware of some of the best practices to follow when adding hover effects to images on your site.
- Don’t go overboard; understanding your audience, your website, and how to maintain a consistent and professional feel is key. Overuse of effects is a common mistake, leaving your website feeling cluttered and jarring.
- Make sure key information isn’t only displayed in hover effects, as mobile users usually will not have a cursor to trigger the effect. Similarly, information should be accessible via keyboard navigation.
- Make sure your hover effects match your website’s aesthetics: opt for consistent color tones, font and text and so on.
- Set your hover effects to require some delay before activating, as if they are triggered too easily it can create a frustrating experience for users navigating your site.
- Avoid effects which impact legibility. Information and visual cues should be clear to your users.
- Ensure your hover effects don’t impact negatively on site performance or page speed.
Enhance your Website with FooGallery Hover Effects
Hover effects can be beneficial for a website, especially considering the increased engagement and boost in conversions that they can bring. More than this, they provide useful visual cues and information for your site visitors.
However, figuring out how to implement these effects can be difficult. We’ve discussed how to do this manually with simple CSS techniques, and with the support of a WP plugin. As we noted, using CSS comes with potential risks and can be harder to implement, so a plugin is generally a safer, easier option.
FooGallery is a reliable, comprehensive plugin that will greatly facilitate the process of adding hover effects to your images galleries, while ensuring a professional, high-quality finish. FooGallery PRO is an excellent choice, considering its extensive features and hover presets. Look for a FooGallery plan that suits your needs and add hover effects to enhance your website.Creating Hover Effects That Make Your Images Stand Out