Looking to improve your WooCommerce store’s user experience? Implementing AJAX (Asynchronous JavaScript and XML) for add to cart functionality can significantly enhance your online store’s performance and customer satisfaction. Instead of disrupting the shopping experience with full page reloads, AJAX lets customers add products to their cart instantly, keeping them engaged and moving through your sales funnel.
By eliminating page refreshes and enabling background server communication, AJAX add to cart functionality can dramatically reduce cart abandonment rates while improving conversion rates through a faster, more streamlined shopping experience.
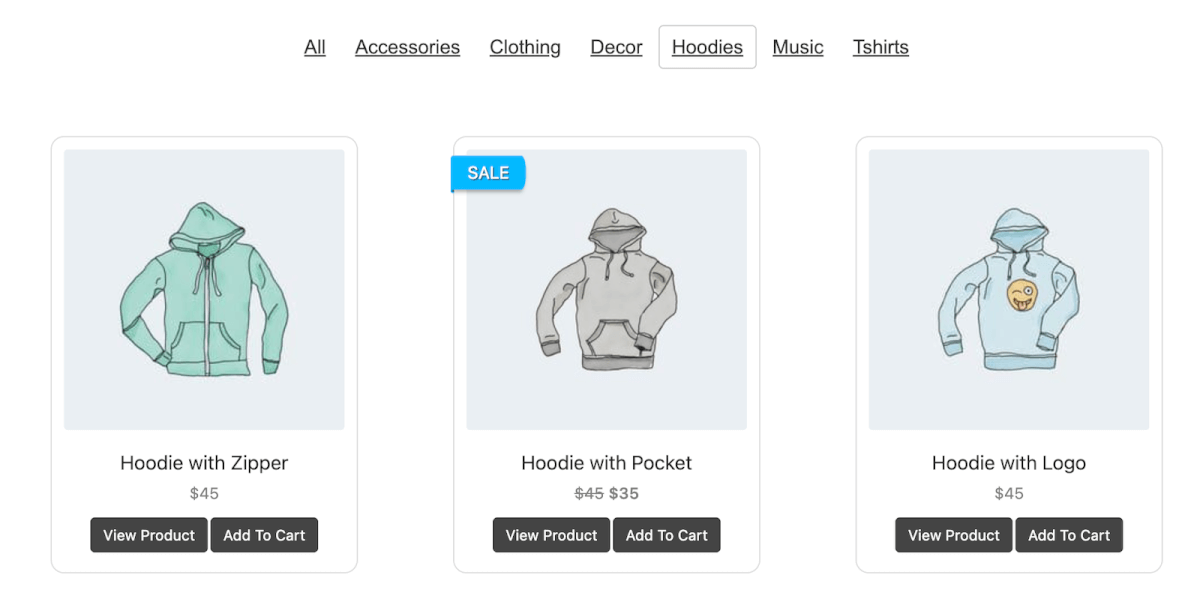
Whether you’re planning to use WooCommerce‘s built-in features, want to implement custom code, or are looking for a plugin solution, this guide will help you create a more professional shopping experience for your customers.
Built-in WooCcommerce Ajax Feature, Activation and Limitations
WooCommerce includes an basic AJAX add to cart feature that works on archive pages (such as your main shop page, category pages, and tag pages). When enabled, customers can add products to their cart without a page refresh – the cart count in your header updates instantly when they click the “add to cart” button.
To activate this feature:
- Navigate to WooCommerce → Settings → Products → General in your WordPress admin.
- Find the “Add to cart behaviour” section.
- Check the box for “Enable AJAX add to cart buttons on archives.”
- Save your changes.
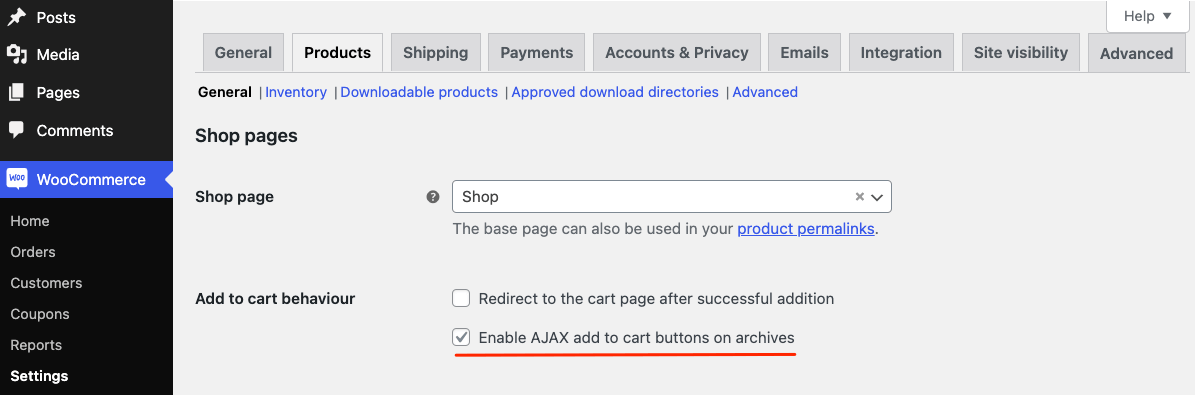
Important notes:
- Most modern WooCommerce-compatible themes support AJAX add to cart out of the box. However, if your theme is not fully WooCommerce-compatible or uses custom product loops, you may need additional code or a child theme adjustment to enable the AJAX functionality correctly.
- By default, WooCommerce loads “cart fragments” (in JavaScript) to automatically update the cart widget and icon. If it’s disabled or removed (often for performance reasons), you may lose the dynamic cart updates unless you use alternative methods to refresh the cart.
While this native functionality is a good starting point, it comes with several important limitations:
- Variable/complex products: Variable products or products requiring customization (e.g., selecting size or color) usually redirect to the single product page for user input, making AJAX adds more complex or requiring additional plugins/shortcodes.
- Caching plugins: Caching plugins or minification settings can sometimes conflict with WooCommerce’s cart fragment scripts, causing the cart not to update in real time. You may need to whitelist or exclude the cart fragments script from certain optimizations.
- Performance overhead: On sites with very high traffic, the cart fragments script may add extra load because it sends AJAX calls on page load to check cart contents. Some store owners choose to disable or optimize it (e.g., using third-party plugins or custom code) to reduce server overhead.
- Limited customization out of the box: The default AJAX behavior mainly covers adding a product to cart and updating the cart count. More advanced AJAX features (like dynamic filtering, infinite scrolling, or advanced product configuration) often require specialized plugins or custom development.
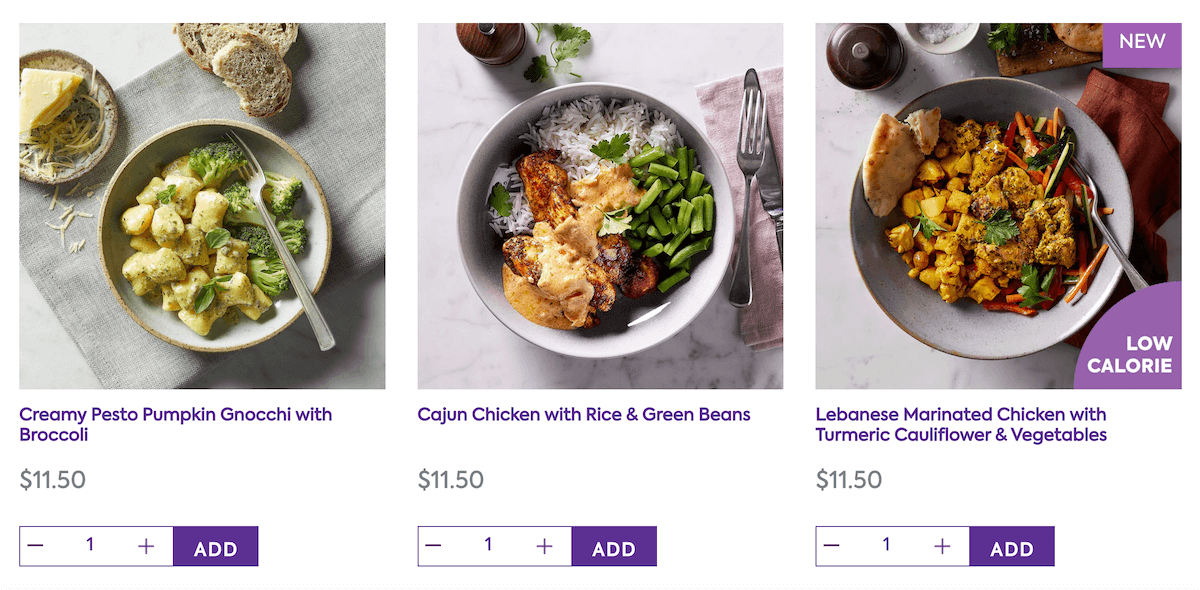
For more robust AJAX cart functionality, you’ll need to consider either a plugin solution or custom code implementation. First, let’s take a look at how to implement AJAX add to cart functionality using code snippets.
How to Implement Woocommerce Ajax Add-to-Cart with Code Snippets
For many stores, the default AJAX add-to-cart functionality is perfectly sufficient and requires no extra coding. But if you have a special layout or you’re pushing the boundaries of what’s possible with WooCommerce, customizing your AJAX implementation can open up new possibilities and provide a smoother and more polished user experience. the WordPress plugin options available to you.
Here is an overview of the basic steps of customizing your AJAX implementation via code:
- Create a custom AJAX endpoint: Use wp_ajax_{action} and wp_ajax_nopriv_{action} hooks to process requests from logged-in and non-logged-in users.
- Add to cart programmatically: Use WooCommerce functions (such as WC()->cart->add_to_cart()) within your AJAX callback.
- Return a response: Send back a JSON response indicating success or failure, along with updated cart totals, messages, or fragment data.
- Handle the response with JavaScript: Update the DOM elements (e.g., cart icon count, mini-cart) based on the data received.
To understand how this implementation works, think of it as a restaurant service system. The JavaScript acts as your waiter, taking orders from customers through their clicks. The PHP code works like the kitchen, processing those orders and ensuring everything is correct. The CSS provides visual feedback, like a buzzer letting customers know their order is being processed.
Let’s get into a simple example. Instead of relying on WooCommerce’s default AJAX settings, we’ll define a custom request flow that we fully control:
- In your child theme’s functions.php file (or a custom plugin), add something like this:
/**
* Register our AJAX add-to-cart actions.
*/
function mytheme_ajax_add_to_cart_init() {
// For logged-in users
add_action( 'wp_ajax_mytheme_ajax_add_to_cart', 'mytheme_ajax_add_to_cart' );
// For guest users
add_action( 'wp_ajax_nopriv_mytheme_ajax_add_to_cart', 'mytheme_ajax_add_to_cart' );
}
add_action( 'init', 'mytheme_ajax_add_to_cart_init' );
/**
* AJAX callback function to handle add to cart.
*/
function mytheme_ajax_add_to_cart() {
// Verify the nonce for security
check_ajax_referer( 'mytheme_ajax_add_to_cart_nonce', 'nonce' );
// Get the product ID from AJAX request
$product_id = isset($_POST['product_id']) ? intval($_POST['product_id']) : 0;
if ( ! $product_id ) {
wp_send_json_error( array( 'message' => __( 'No product ID found.', 'mytheme' ) ) );
}
// Add the product to the cart
$added = WC()->cart->add_to_cart( $product_id );
if ( $added ) {
// Optionally, update mini-cart or total fragments
// WooCommerce has built-in functions to generate cart fragments if needed
// Prepare the success response
$response = array(
'message' => sprintf( __( '%s has been added to your cart.', 'mytheme' ), get_the_title( $product_id ) ),
'cart_count' => WC()->cart->get_cart_contents_count(),
'cart_subtotal' => strip_tags( WC()->cart->get_cart_subtotal() ),
);
wp_send_json_success( $response );
} else {
wp_send_json_error( array( 'message' => __( 'Could not add product to cart.', 'mytheme' ) ) );
}
}
Code language: PHP (php)
2. You’ll need a small script (inline or in a separate .js file) to capture the click event and send data to your PHP endpoint.
In your child theme or plugin’s JS do something like:
(function($) {
$(document).ready(function() {
$('.mytheme-add-to-cart-btn').on('click', function(e) {
e.preventDefault();
// Retrieve product ID from a data attribute on the button
let productId = $(this).data('product-id');
let nonce = mytheme_ajax_add_to_cart.nonce; // Localized nonce
$.ajax({
url: mytheme_ajax_add_to_cart.ajax_url, // Localized AJAX URL
type: 'POST',
dataType: 'json',
data: {
action: 'mytheme_ajax_add_to_cart',
product_id: productId,
nonce: nonce
},
success: function(response) {
if ( response.success ) {
// Update cart icon, subtotal, show success message, etc.
$('.cart-count').text(response.data.cart_count);
$('.cart-subtotal').text(response.data.cart_subtotal);
alert(response.data.message);
} else {
// Handle error
alert(response.data.message);
}
},
error: function() {
alert('Something went wrong with the AJAX call.');
}
});
});
});
})(jQuery);
Code language: JavaScript (javascript)
3. In your functions.php (or plugin file), ensure you enqueue and localize the above script:
function mytheme_enqueue_scripts() {
wp_enqueue_script(
'mytheme-ajax-cart',
get_stylesheet_directory_uri() . '/js/mytheme-ajax-cart.js',
array('jquery'),
'1.0',
true
);
wp_localize_script(
'mytheme-ajax-cart',
'mytheme_ajax_add_to_cart',
array(
'ajax_url' => admin_url('admin-ajax.php'),
'nonce' => wp_create_nonce('mytheme_ajax_add_to_cart_nonce')
)
);
}
add_action('wp_enqueue_scripts', 'mytheme_enqueue_scripts');
Code language: PHP (php)
Once this is set up, any button with the class mytheme-add-to-cart-btn and a data-product-id=”1234″ attribute will trigger an AJAX add-to-cart when clicked.
This code creates a custom AJAX “Add to Cart” process in WooCommerce. On the PHP side, it registers an AJAX action for both logged-in and guest users, verifies a security nonce, adds the product to the cart, and returns a JSON response. On the JavaScript side, it listens for clicks on a button that includes a product ID, sends that ID (along with the nonce) to the PHP endpoint via AJAX, and then updates the cart count and subtotal on success – without requiring a full page reload. The script localization in functions.php simply provides the JavaScript file with the correct admin-ajax.php URL and security nonce for secure requests.
Implementation Tips
Testing and monitoring your AJAX cart implementation is crucial for providing a smooth shopping experience. Start by testing basic scenarios like adding simple and variable products, then progress to more complex situations like multiple rapid additions. Always test with a simulated slow internet connection to ensure your feedback mechanisms work effectively.
- Mobile Testing: Pay special attention to touch events and visual feedback. Ensure your cart updates and loading indicators are clearly visible on smaller screens, and test common mobile behaviors like rapid tapping or swipe interactions. A loading spinner or visual feedback should always be visible above the fold.
- Performance Monitoring: Set up basic analytics tracking to understand how your AJAX cart performs. Use Google Analytics to track successful cart additions and cart abandonment rates before and after implementation. Tools like Hotjar can provide valuable insights into how customers interact with your add-to-cart buttons and help identify potential friction points in the process.
- A/B Testing: Run simple tests to find what works best for your customers. Compare different loading indicators (like spinners versus progress bars), test message placement (such as notifications next to the button or in a slide-in panel), and try various mini-cart animations (from subtle number updates to quick preview drawers). Use Google Analytics to track which combinations lead to more completed purchases rather than just cart additions.
- Error Handling: Always provide clear, actionable feedback to your customers. Instead of technical error messages, use friendly language that guides users toward solutions. For example, rather than “Variation ID invalid,” say “Please select your preferred size and color before adding to cart.”
- Security Measures: When implementing AJAX cart functionality, always prioritize security to protect your store and customers. Start by validating all user inputs both on the browser and server side – never trust data coming directly from the customer’s browser. Use WordPress’s built-in security features like nonces to verify legitimate requests, and implement basic rate limiting to prevent rapid-fire cart additions that could strain your server. Most importantly, test your security measures regularly by trying to add invalid products or manipulate quantities, ensuring your store remains secure while providing a smooth shopping experience.
While custom code provides maximum flexibility, it requires regular maintenance to stay secure and compatible with WooCommerce updates. For most store owners, using well-maintained plugins offers a more reliable and secure no-code solution that automatically adapts to WooCommerce changes.
Top 5 AJAX Add to Cart Plugins for WooCommerce
1. FooGallery PRO Commerce
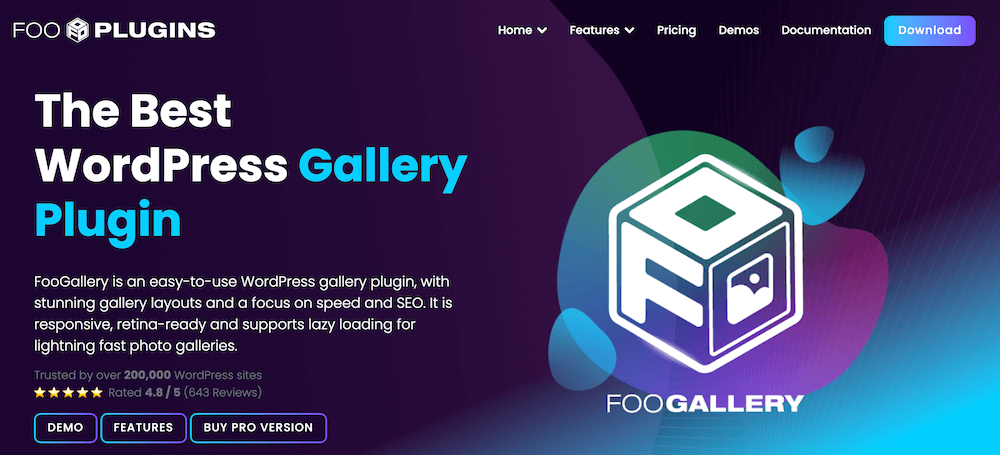
FooGallery PRO Commerce is a paid plugin that combines product gallery functionality with AJAX cart features, offering:
- Add to Cart and redirect to checkout: Users are redirected to the checkout page after adding an item.
- AJAX-enabled add to cart buttons that can be placed within product galleries.
- Four customizable cart behaviors:
- Add to Cart (AJAX): This adds items to the cart without having to reload the page.
- Add to Cart and refresh page: Items are added to the cart and the page refreshes.
- Add to Cart and redirect to cart: Users are redirected to the cart page after adding an item.
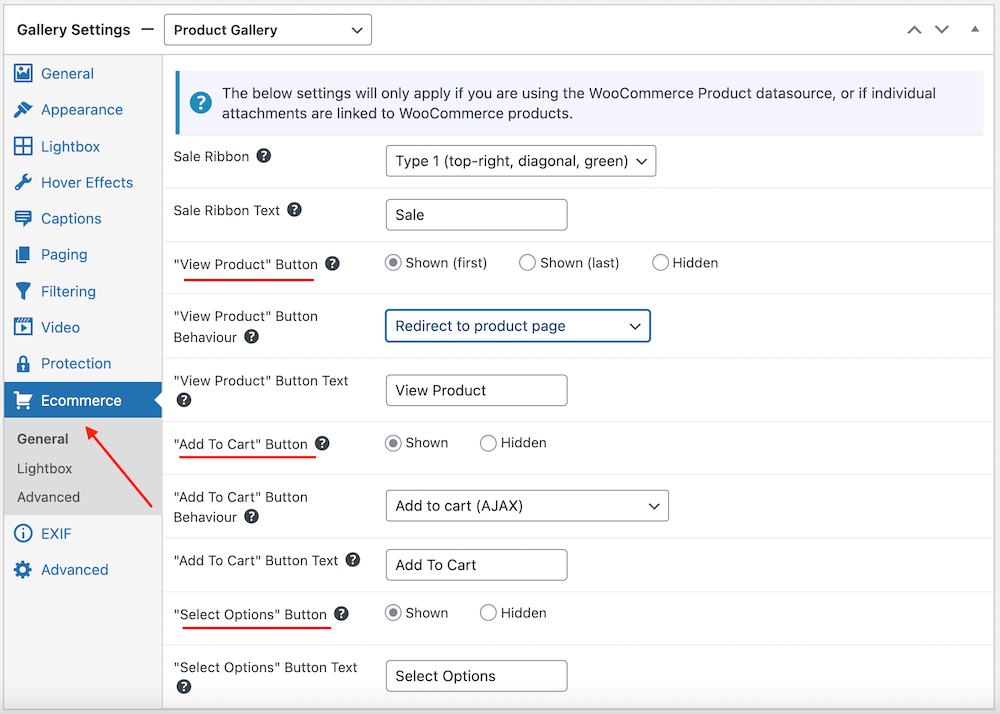
- Support for variable products with a “Select Options” button.
- Integration with custom gallery layouts.
- Ecommerce-related features such as custom Add to cart buttons, file downloads, and sale ribbons.
Limitations:
- Premium pricing may be a barrier for small stores.
- AJAX functionality not available on Single Product Pages.
- Requires additional setup to create and place galleries.
Best for: Store owners who want to combine product galleries with AJAX cart functionality, especially useful for creating custom product showcases outside the standard shop pages.
The Best WordPress Gallery Plugin
FooGallery is an easy-to-use WordPress gallery plugin, with stunning gallery layouts and a focus on speed and SEO.
2. Ajax add to cart for WooCommerce by QuadLayers
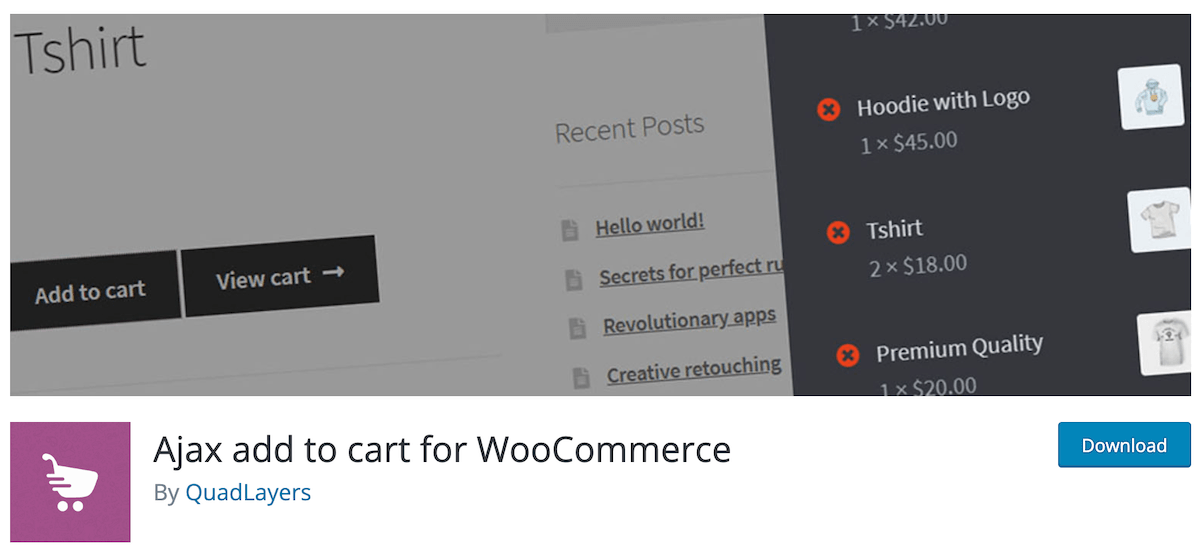
Ajax add to cart for WooCommerce by QuadLayers is a free plugin that focuses on core AJAX functionality:
- Adds AJAX capability to all add-to-cart buttons.
- Works with both simple products and variations.
- Optimized for performance with minimal server load.
- Simple setup with no configuration required.
Limitations:
- Limited customization options.
- No advanced features like cart previews.
- May conflict with some theme customizations.
Best for: Store owners looking for a lightweight, straightforward AJAX solution without additional features.
3. WooCommerce Builder For Divi
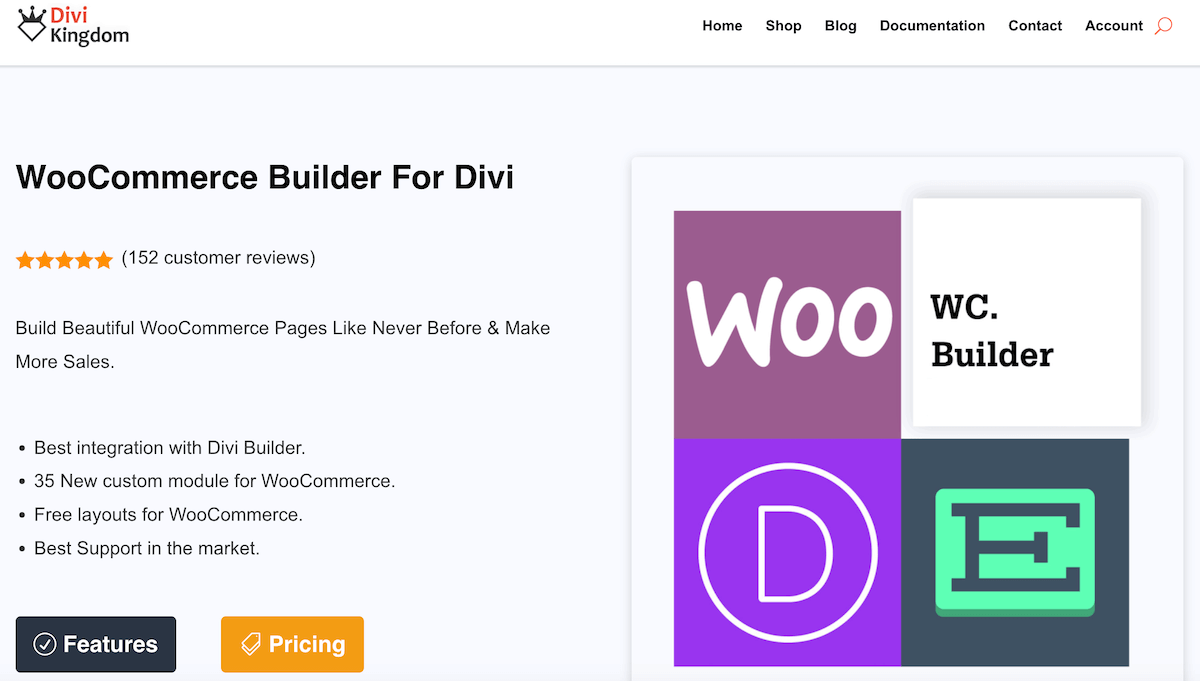
While primarily a Divi extension, WooCommerce Builder For Divi plugin offers robust AJAX cart features:
- Full AJAX integration for cart operations.
- Custom cart and empty cart page layouts.
- Configurable add-to-cart notifications and button behaviors.
- Mini cart functionality with AJAX updates.
Limitations:
- Only works with Divi theme, making it unsuitable for stores using other themes.
- Significant investment required (both Divi and plugin costs).
- It takes longer to comprehend as you need to understand Divi’s page builder first.
- Resource-heavy compared to standalone AJAX solutions.
Best for:Divi theme users who want integrated AJAX functionality within their builder interface.
4. WPC Ajax Add to Cart for WooCommerce
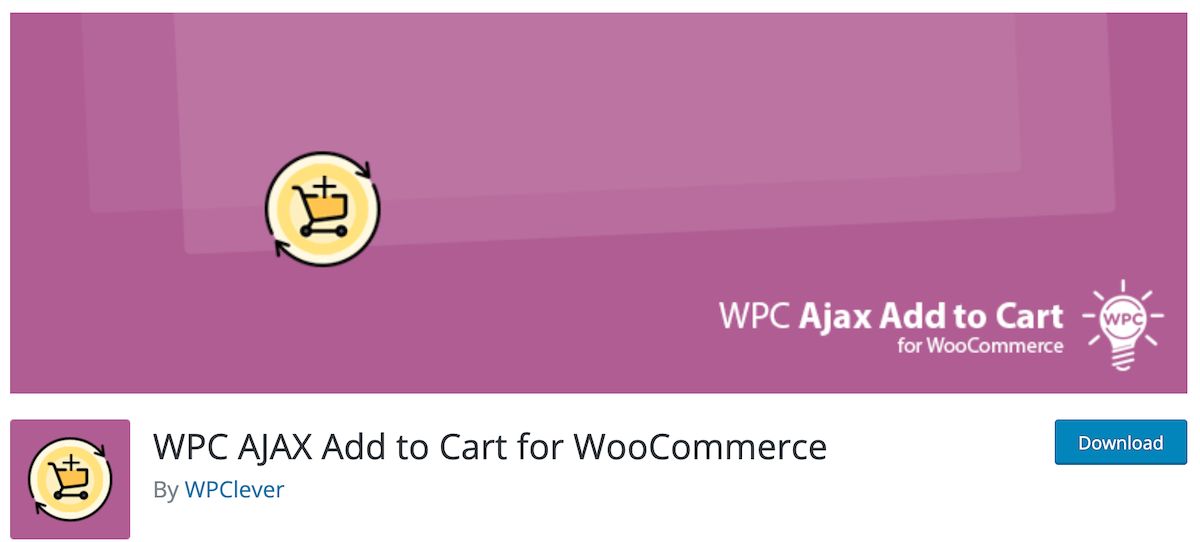
WPC Ajax Add to Cart for WooCommerce is a free plugin that provides comprehensive AJAX cart functionality:
- Easy to install and use.
- Works on simple and variable products.
- Compatible with most WordPress themes and WooCommerce plugins.
Limitations:
- Full functionality requires additional WPClever plugins like Fly Cart.
- Some features are restricted to premium versions.
Best for: Store owners who are already using or planning to use other WPClever plugins, as it works best when integrated with their ecosystem.
5. Floating Sticky Cart for WooCommerce
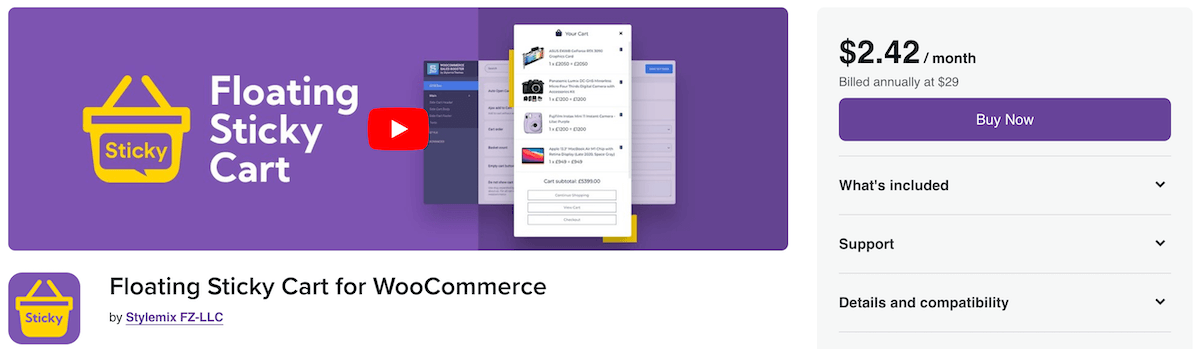
Floating Sticky Cart for WooCommerce combines AJAX cart functionality with a floating cart interface:
- Persistent cart visibility across all pages.
- AJAX-powered cart updates.
- Customization options.
- Built-in cross-sell and upsell features.
- Coupon code application support.
- Paypal integration for direct check out.
Limitations:
- Requires careful consideration for theme compatibility.
Best for: Stores wanting to combine AJAX functionality with an always-visible cart interface.
Boost your store’s conversion rate with FooGallery’s AJAX Add to Cart features
While custom code and basic plugins can add AJAX functionality to your cart buttons, FooGallery PRO Commerce offers a complete solution that goes beyond simple cart updates. Instead of just modifying existing buttons, it enables you to create strategic product galleries throughout your store displaying Add to Cart Buttons each equipped with AJAX add-to-cart functionality.
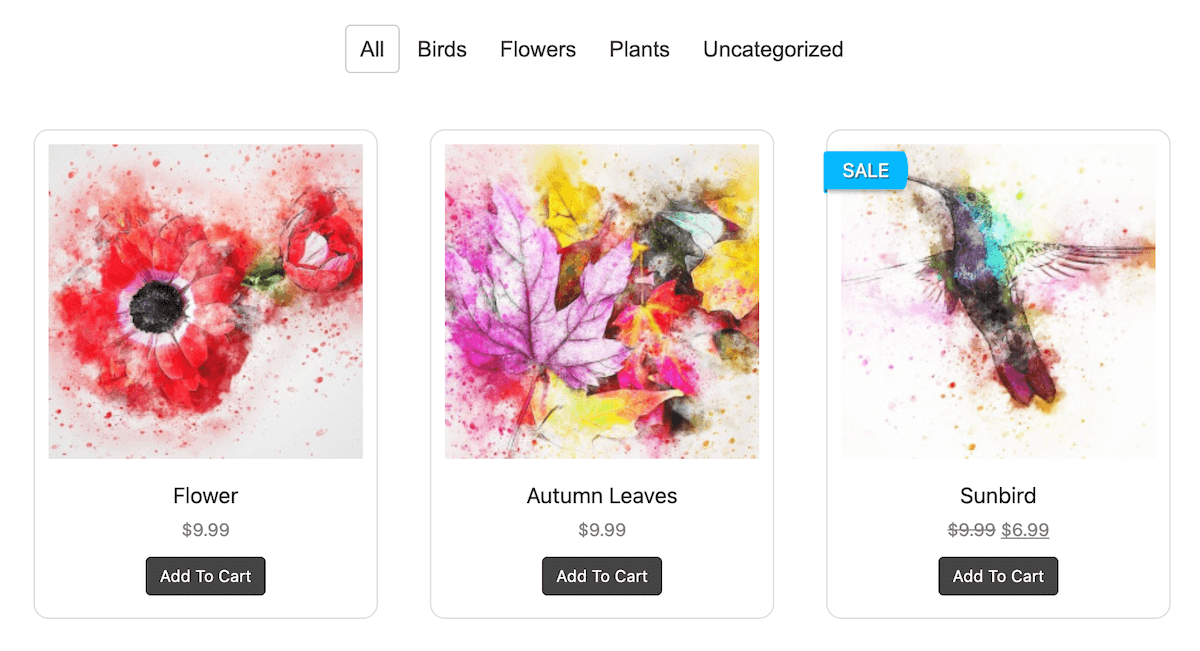
Unlike other solutions we’ve explored, FooGallery PRO Commerce combines two crucial elements for ecommerce success: enhanced product visibility and frictionless purchasing. By displaying your products in customizable galleries at key points in your store, you can showcase more items to potential customers. When they find something they like, the built-in AJAX cart functionality ensures they can continue browsing without interruption.
I’ve been using this gallery for over a year now and love it! Super easy to use, and it looks wonderful – exactly what I needed.
Annemarie Horne
Ready to enhance your WooCommerce store with professional-grade product galleries and seamless AJAX cart integration? Try FooGallery PRO Commerce today.